Using the Swift SMS API is easy!
Swift SMS Gateway® connects your business text messaging using a simple SMS, or MMS API call from our website to cell phones globally.
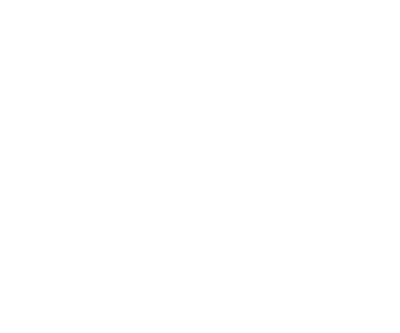
1) Get an Account Key
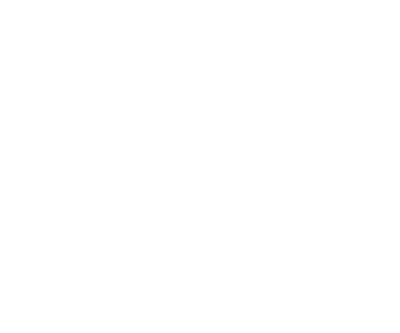
2) Pick Your Code
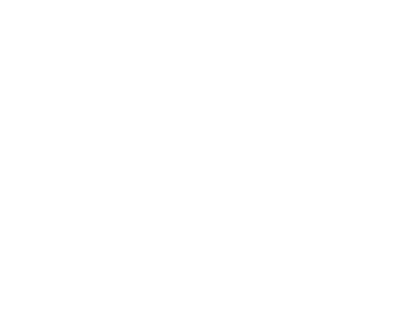
3) Send Your Message
API Integration
Use the power of the Swift SMS Gateway® API to send SMS and MMS. It’s fast and easy. We provide a full library of programming documentation with popular languages that include RESTful and Legacy examples. Get Your Code Now!
// uses JQuery library
var postUrl = “https://secure.smsgateway.ca/services/message.svc/”
}+ accountKey + “/” + destinationNumber
var body = JSON.stringify({
MessageBody: “Message Body”
});
$.ajax({
url: postUrl,
method: “POST”,
contentType: “application/json;charset=UTF-8”,
data: body
}).done(function(response) {
alert(response);
}).error(function (xhr, textStatus, errorThrown) {
alert (xhr.responseText);
});
<?php
// using SOAP Module – http://ca3.php.net/soap
class SMSParam {
public $CellNumber;
public $AccountKey;
public $MessageBody;
}
$client = new SoapClient(‘https://secure.smsgateway.ca/sendsms.asmx?WSDL’);
$parameters = new SMSParam;
$parameters -> CellNumber = destinationNumber;
$parameters -> AccountKey = accountKey;
$parameters -> MessageBody = “This is a demonstration of SMSGateway.ca using PHP5.”;
$Result = $client->SendMessage($parameters);
?>
‘Using Service Reference (SOAP)
Using client = New SwiftSMS.SendSMSSoapClient
Dim response = client.SendMessage(destinationNumber, messageBody, accountKey)
End Using
‘Using Webclient (REST)
Dim url = String.Format(“https://secure.smsgateway.ca/services/message.svc/{0}/{1}”, accountKey, destinationNumber)
Dim body = String.Format(“{{ “”MessageBody””: “”{0}””, “”Reference”” : “”{1}”” }}”, messageBody, reference)
Using wClient = New Net.WebClient
wClient.Encoding = New UTF8Encoding()
wClient.Headers.Add(“content-type”, “application/json”)
Dim wResponse = wClient.UploadString(url, body)
End Using
Simple HTTP GET:
curl “https://secure.smsgateway.ca/SendSMS.aspx?CellNumber=[destinationNumber]&AccountKey=[accountKey]&MessageBody=[messageBody]”
HTTP POST:
curl -H “Content-Type: application/json” -X POST \
“https://secure.smsgateway.ca/services/message.svc/[accountKey]/[destination]” \
––data “{ \”MessageBody\”: \”[messageBody]\” }”
// Service Reference / SOAP
using (var client = new SwiftSMS.SendSMSSoapClient())
{
string response = client.SendMessage(destinationNumber,
messageBody, accountKey);
}
// Web Client / REST
dynamic body = new ExpandoObject();
body.MessageBody = “Message Body”;
var url = string.Format(“https://secure.smsgateway.ca/services/message.svc/{0}/{1}”,
accountKey, destinationNumber);
using (var wClient = new System.Net.WebClient())
{
wClient.Encoding = Encoding.UTF8;
wClient.Headers.Add(“content-type”, “application/json”);
string response = wClient.UploadString(url,
Newtonsoft.Json.JsonConvert.SerializeObject(body));
}